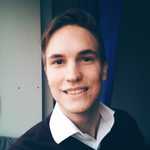
GustavBylund
+46 (0)73 026 26 86hello@gustavbylund.semaisthoAutofill Squirdle filters
Squirdle is a fun wordle-like with a Pokémon theme. This bookmarklet makes typing in the filters quicker :)
Bookmarklet
Drag the following link to your bookmark bar!
Autofill SquirdleOr add this code to a bookmark:
javascript:!function(){const e=document.querySelector("#guess"),r=Array.from(document.querySelectorAll("#guesses [id^=guess]")).map(e=>{return{emojis:Array.from(e.querySelectorAll(".emoji")).map(e=>e.src.match(/\/(\w+)\.png$/)[1]),values:pokedex[e.querySelector(".guess").textContent]}}),n=r.reduce((n,t)=>(t.emojis.forEach((e,r)=>{"wrong"==e?(null==n[r][e]&&(n[r][e]=new Set),n[r][e].add(t.values[r]||"None")):("correct"===e||null==n[r][e]||"up"===e&&t.values[r]>n[r][e]||"down"===e&&t.values[r]<n[r][e]||"wrongpos"===e)&&(n[r][e]=t.values[r])}),n),[{},{},{},{},{}]),t=["gen","type","type","height","weight"],o=Array.from(new Set(n.map((e,n)=>{if("type"!==t[n])return null!=e.correct?[t[n]+":"+e.correct]:Object.entries(e).map(([e,r])=>{switch(e){case"up":return t[n]+">"+r;case"down":return t[n]+"<"+r}}).flat();{const r=[];return null!=e.wrongpos&&r.push(t[n]+":"+(e.wrongpos||"None")),null!=e.correct&&r.push(t[n]+":"+(e.correct||"None")),e.wrong&&r.push(...Array.from(e.wrong).map(e=>t[n]+"!"+e)),r}}).flat()));e.value=o.join(" "),e.dispatchEvent(new Event("input",{bubbles:!0,cancelable:!0}))}();
Full code available below:
type GuessMap = {
correct?: string | number
wrongpos?: string
wrong?: Set<string>
up?: number
down?: number
}
declare const pokedex: Record<string, [number, string, string, number, number]>
;(function () {
const guessEl: HTMLInputElement = document.querySelector('#guess')
const guesses = Array.from(
document.querySelectorAll('#guesses [id^=guess]'),
).map((el) => {
const emojis = Array.from(
el.querySelectorAll<HTMLImageElement>('.emoji'),
).map((em) => em.src.match(/\/(\w+)\.png$/)[1])
const values = pokedex[el.querySelector('.guess').textContent]
return { emojis, values }
})
const reduced = guesses.reduce(
(acc, curr) => {
curr.emojis.forEach((emoji, index) => {
if (emoji == 'wrong') {
if (acc[index][emoji] == undefined) {
acc[index][emoji] = new Set()
}
acc[index][emoji].add((curr.values[index] as string) || 'None')
} else if (emoji === 'correct' || acc[index][emoji] == undefined) {
acc[index][emoji] = curr.values[index]
} else if (emoji === 'up' && curr.values[index] > acc[index][emoji]) {
acc[index][emoji] = curr.values[index] as number
} else if (emoji === 'down' && curr.values[index] < acc[index][emoji]) {
acc[index][emoji] = curr.values[index] as number
} else if (emoji === 'wrongpos') {
acc[index][emoji] = curr.values[index] as string
}
})
return acc
},
<GuessMap[]>[{}, {}, {}, {}, {}],
)
const names = ['gen', 'type', 'type', 'height', 'weight'] as const
const values = Array.from(
new Set(
reduced
.map((res, index) => {
if (names[index] === 'type') {
const arr = []
if (res.wrongpos != undefined) {
arr.push(`${names[index]}:${res.wrongpos || 'None'}`)
}
if (res.correct != undefined) {
arr.push(`${names[index]}:${res.correct || 'None'}`)
}
if (res.wrong) {
arr.push(
...Array.from(res.wrong).map(
(type) => `${names[index]}!${type}`,
),
)
}
return arr
} else if (res.correct != undefined) {
return [`${names[index]}:${res.correct}`]
} else {
return Object.entries(res)
.map(([k, v]) => {
switch (k) {
case 'up':
return `${names[index]}>${v}`
case 'down':
return `${names[index]}<${v}`
}
})
.flat()
}
})
.flat(),
),
)
guessEl.value = values.join(' ')
guessEl.dispatchEvent(
new Event('input', {
bubbles: true,
cancelable: true,
}),
)
})()